Lab Solutions:
- Lab 1:
TP1.zip
TP1.tar.xz
- Lab 2:
TP2.zip
TP2.tar.xz
- Lab 3:
TP3.zip
TP3.tar.xz
- Lab 4:
TP4.zip
TP4.tar.xz
- Lab 5:
TP5.zip
TP5.tar.xz
FAQ Quick Jump:
- Where can I get access to the course handouts and lab instructions ?
- I'm new to linux, how can I get started ?
- What text editor should I use to code ?
- How to write a generic
Makefile
? - How to build my code without using the terminal ? (updated for codeblocks)
- How to work on my personal computer ?
- How to work remotely from home ?
- How to download my code from the school server ?
- How to document my code with
doxygen
? - How to debug
segfaults
and memory leaks ? - How to debug my code ?
- Where can I find a free book to learn C++ ? (new)
Where can I get access to the course handouts and lab instructions ?
Everything is available on Laurence Pierre personal website.I'm new to linux, how can I get started ?
Here is a short article on how to use the linux terminal and the basics to work with directories and files.What text editor should I use to code ?
Please do not usegedit
for C++
projects, you will miss all modern features a decent text editor should have.
I would recommend one of those three editors:
Atom is already installed on school computers, you can launch it with the following command in a terminal:
- atom
Here is an example of what you will get for free with Atom
(Tab-completion):
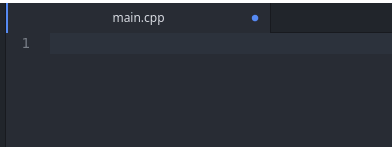
Of course if you are already using vim
or emacs
, you can stick with it !
How to write a generic Makefile
?
Here is a sample Makefile
that should work for every exercices.
- SRC=src
- INC=include
- BIN=bin
- SRCS=$(wildcard ${SRC}/*.cpp)
- OBJS=$(patsubst ${SRC}/%.cpp, ${BIN}/%.o, ${SRCS})
- TARGET_BINARY=${BIN}/main #[replace by target_binary_name]
- CXX ?= g++ # or clang++
- CXXFLAGS += -I${INC} -std=c++14 -pedantic
- CXXFLAGS += -Wall -Wextra -Wno-unused
- all: ${TARGET_BINARY}
- ${TARGET_BINARY} : ${OBJS}
- ${CXX} $^ -o $@
- ${BIN}/%.o: ${SRC}/%.cpp
- ${CXX} ${CXXFLAGS} -c $< -o $@
- .PHONY: clean
- clean:
- rm -f ${OBJS}
- rm -f ${TARGET_BINARY}
This Makefile
enables C++14
and extra warnings to help you to debug your code.
It should be placed in the root folder that contains src
, include
and bin
(unlike the one in the first lab).
Example usage:
- make
- make clean
- CXX=clang++ make
- CXXFLAGS=-Wsign-compare make
How to build my code without using the terminal ?
Every editor should provide a way to build and run external programs.Here is how to call
make
within some editors:
-
Atom:
- Go to
Edit > Preferences > +Install
- Search and install the following packages (and dependencies):
- build
- build-make-file
- Use F7 to choose build target (all or clean for example).
- Use F9 to build.
- Go to
-
Sublime Text:
- Go to
Tools > Build System
and tickMake
. - Open the
Makefile
in sublime and make it active. - Use Ctrl+b to build.
- Go to
-
Vim:
- Make is a builtin feature of
vim
, just use Esc:make
- Make is a builtin feature of
-
Codeblocks
- Create a C++ console project:
File > New > Project > Console application
- Click on
Go
, selectC++
, click onNext
. - Choose a project title, for example
exercise1
. - Carefully check
'Folder to create project in'
and'Resulting filename'
. - Click on
Next
. - Select
GNU GCC Compiler
and click onFinish
.
- Import
.cpp
and.h
files if any:- Rightclick on project name and use
Add files
. - You can create empty files by using
File > New > Empty file
.
- Rightclick on project name and use
- Add your custom
Makefile
as build target:- Rightclick on project name and click on
Properties
. - In
Project settings
under theMakefile
entry, tick'This is a custom Makefile'
. - In
Build targets
, click onAdd
, enter'all'
and clickOk
. -
Delete the
Debug
andRelease
build targets. - Modify the
'Type'
entry of your new target toConsole application
. - Modify the
'Output filename'
entry of your new target to the name of the output executable given by yourMakefile
(bin/ex1_date
for the first exercice of first lab). -
Finally click on
Ok
to close theProject properties
. - Execute the program by using a terminal.
- Rightclick on project name and click on
- Modify the
Makefile
:- Rename the
clean
rule tocleanall
.
- Rename the
- Create a C++ console project:
How to work on my personal computer ?
The only dependencies for the labs aremake
and a C++
compiler (gcc or clang).
You need root access on your machine to be able to install them.
- Mac OS X:
You need to install
Xcode
and thecommand line tools
, see this link. - Linux (ubuntu, arch, debian, centos, ...):
- sudo apt-get update
- sudo apt-get install make g++ clang
- Windows 10:
You need to install
WSL
(Windows Linux Subsystem
), this will require~700MB
of free space.- First fully update your Windows 10.
- Got to the
Microsoft Store
and search forUbuntu 18.04 LTS
. - Install it.
- Open the
Windows Powershell
with administrative rights.
To open it as administrator look for it with the search bar and then use Ctrl + Shift + Left Click.
Once launched, enter his command to enable the linux subsystem:- Enable-WindowsOptionalFeature -Online -FeatureName Microsoft-Windows-Subsystem-Linux
- If the command fails, this means you did not run the
powershell
as administrator. - You will be prompted to reboot, juste type Y to reboot.
- Launch
Ubuntu 18.04 LTS
, you will have to choose alogin
and apassword
. - You will get access to a linux terminal.
- Fully upgrade the linux subsystem and install what we need with the following commands:
- sudo apt-get update
- sudo apt-get full-upgrade
- sudo apt-get install make g++ clang
- From here you can use
ssh
andscp
to get your code from the school server. - Create a shortcut to your linux home folder:
- Navigate to
C:\Users\[windows username]\AppData\Local\Packages\ CanonicalGroupLimited.Ubuntu18.04onWindows_[...]\LocalState\rootfs\home
- Right click on the folder named as the linux login you just chose and send it to your
Desktop
(this will create a shortcut to your linux home folder on your Windows desktop).
- Navigate to
- Open you project folder in your favourite text editor for
Windows
(Atom
for example) by using this shortcut. - Use
make
and execute binaries in the linux terminal.
- g++ --version
- clang++ --version
- make --version
How to work remotely from home ?
You can access the school servermandelbrot
from anywhere by using the ssh
protocol.
- If using Windows, install an use a GUI ssh client
(for example
PuTTY
). - Connect to the server
mandelbrot.e.ujf-grenoble.fr
with your usual logins.
- ssh -X username@mandelbrot.e.ujf-grenoble.fr
-X
option will grant you a GUI environment on the remote server, this means that
you will be able to launch graphical applications as if you were using the
computer locally.
Update:
Atom
does not work on mandelbrot
, you can only use
gedit
, emacs
and vim
(and it is really slow).
How to download my code from the school server ?
You usescp
(secure copy protocol) or sftp
(ssh file transfer protocol) from and to the school server.
- If using Windows, install and use a GUI
sftp
client (for exampleFileZilla
). - Connect to
mandelbrot.e.ujf-grenoble.fr
with your usual logins.
~/Documents/CPP/TP1
(on the school server) and you want to get a copy on your personal computer,
just execute this command on your machine:
- scp -r username@mandelbrot.e.ujf-grenoble.fr:~/Documents/CPP/TP1 .
How to document my code with doxygen
?
- In your project folder (where you have the
src
andinclude
folders for example), create adocs
folder, aREADME.md
file and aDoxyfile
with the following commands:- mkdir docs
- touch README.md
- doxygen -g
bin docs Doxyfile include Makefile README.md src
- Modify the
Doxyfile
with your favorite text editor and edit the following variables:PROJECT_NAME
: Project namePROJECT_BRIEF
: Project descriptionOUTPUT_DIRECTORY
:./docs
INPUT
:./README.md ./src ./include
USE_MDFILE_AS_MAINPAGE
:./README.md
EXTRACT_PRIVATE
:YES
- Add the following rules to your
Makefile
:- DOCS=docs
- doc:
- doxygen
- html: doc
- $(BROWSER) ${DOCS}/html/index.html
- pdf: doc
- $(MAKE) -C ${DOCS}/latex
- evince ${DOCS}/latex/refman.pdf
make doc
will build the documentation usingdoxygen
and compile the generated latex files.make html
will open your documentation in your browser.make pdf
will open your documentation with a pdf viewer.
- Try it, you should get a basic documentation with the title of your project.
- Add
doxygen
comments in your code as documented here.
You can put doxygen documentation in both your headers and your source code files. -
The main page of your documentation is determined by the
markdown
file
README.md
.
Here is a sampleREADME.md
for the first exercice of lab 2:- Daily trip price calculator {#mainpage}
- ===========================
- About
- -----
- This amazing program computes the average price of a trip spanning
- between two user given dates.
- Build
- -----
- You will need a C++14 compatible compiler.
- To build this code you just need to do the following:
- mkdir bin
- make -jN
- where N is the number of available processes.
- Documentation
- -------------
- To build this documentation you can use the following commands:
- * `make doc`: will build the documentation.
- * `make html`: open the `html` documentation in your browser.
- * `make pdf`: open the `pdf` documentation using `evince`.
- Program usage
- -------------
- `./bin/ex1_trip [sday] [smonth] [syear] [eday] [emonth] [eyear]`
- Quick documentation overview:
- -----------------------------
- Here are the two main classes used by this project:
- 1. #Date ([date.h](@ref date.h) and [date.cpp](@ref date.cpp)):
- Implementation of basic dates functionalities
- 2. #Trip ([trip.h](@ref trip.h) and [trip.cpp](@ref trip.cpp)):
- Implementation of trips.
- Some utility function are hidden in [utils.h](@ref utils.h)
- and [utils.cpp](@ref utils.cpp).
- The main function is hidden in [main.cpp](@ref main.cpp).
How to debug segfaults
and memory leaks ?
On linux you can use valgrind
:
- Add the debug flag
-g
to yourCXXFLAGS
variable in yourMakefile
. - Recompile your whole project (clean and build):
- make clean && make
- Launch your programm with
valgrind
- valgrind [binary] [args]
How to debug my code ?
On linux you can usegdb
:
- Add the debug flag
-g
to yourCXXFLAGS
variable in yourMakefile
. - Recompile your whole project (clean and build):
- make clean && make
- Debug your programm with
gdb
- gdb -tui --args [binary] [args]
- Type
run
to execute your program.